LeetCode # 989
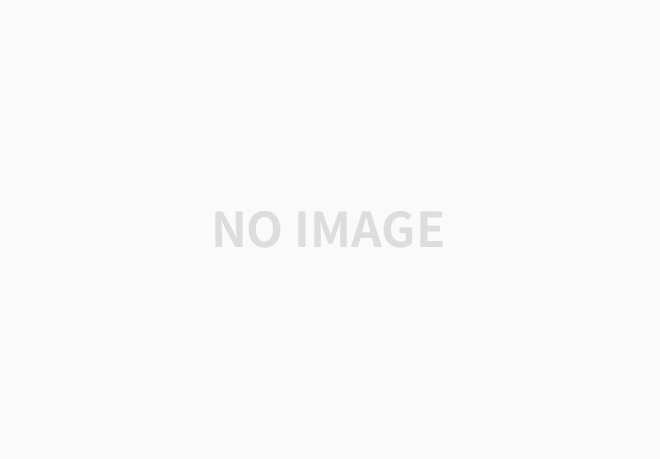
Example 1:
Input:
num = [1,2,0,0], k = 34
Output: [1,2,3,4]
Explanation: 1200 + 34 = 1234
Example 2:
Input:
num = [9,9,9,9,9,9,9,9,9,9], k = 1
Output: [1,0,0,0,0,0,0,0,0,0,0]
Explanation: 9999999999 + 1 = 10000000000
Constraints:
- 1 <= num.length <= 104
- 0 <= num[i] <= 9
- num does not contain any leading zeros except for the zero itself.
- 1 <= k <= 104
Solution #1 (3ms)
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
class Solution {
public List<Integer> addToArrayForm(int[] num, int k) {
List<Integer> result = new ArrayList<>();
int i = num.length - 1;
int sum = 0;
while (i >= 0 || k > 0) {
if (i >= 0) {
sum += num[i];
i--;
}
if (k > 0) {
sum += k % 10;
k /= 10;
}
result.add(sum % 10);
if (sum > 9) {
if (i < 0 && k == 0) result.add(1);
sum /= 10;
} else {
sum = 0;
}
}
Collections.reverse(result);
return result;
}
}
Result #1
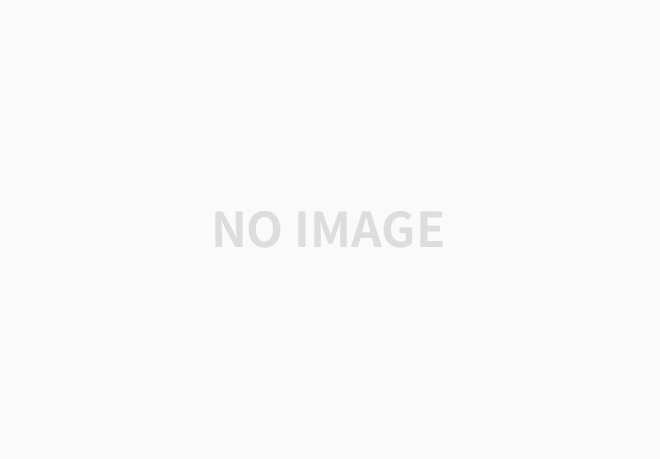
💡 직관적인 풀이 방법, 하지만 리팩토링이 필요하다.
불필요한 코드가 많다. 조건문을 최소화하고, Collections 함수를 사용하지 않을 방법을 찾아보자.
Solution #2 (3ms)
import java.util.LinkedList;
import java.util.List;
class Solution {
public List<Integer> addToArrayForm(int[] num, int k) {
LinkedList<Integer> list = new LinkedList<>();
int i = num.length - 1, sum = k;
while (i >= 0 || sum > 0) {
if (i >= 0) sum += (num[i]);
list.addFirst(sum % 10);
sum /= 10;
i--;
}
return list;
}
}
Result #2
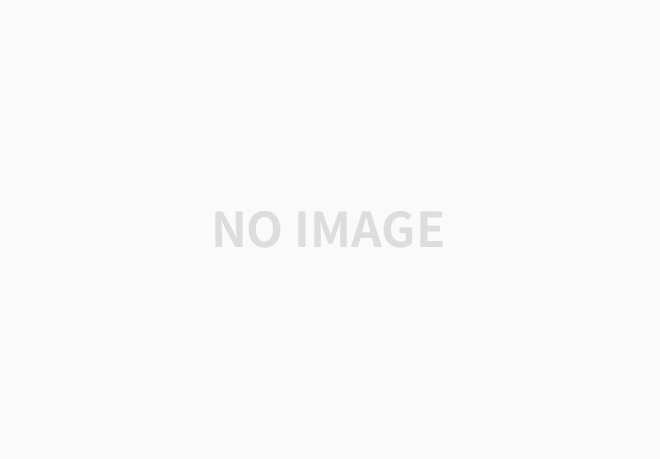
💡 ArrayList에서 LinkedList로 자료형 변경
LinkedList의 addFirst() 메소드를 이용하여 Collections 함수는 제거할 수 있었지만 그만큼 Memory Usage가 증가했다.
More Algorithm!
👇👇
github.com/ggujangi/ggu.leet-code
ggujangi/ggu.leet-code
LeetCode, Java. Contribute to ggujangi/ggu.leet-code development by creating an account on GitHub.
github.com
출처 : leetCode
'알고리즘 > LeetCode' 카테고리의 다른 글
[Java] LeetCode 561 : Array Partition I (0) | 2021.05.14 |
---|---|
[Java] LeetCode 441 : Arranging Coins (0) | 2021.05.14 |
[Java] LeetCode 415 : Add Strings (0) | 2021.05.12 |
[Java] LeetCode 258 : Add Digits (0) | 2021.05.12 |
[Java] LeetCode 67 : Add Binary (0) | 2021.05.12 |